TypeScript autocomplete with arbitrary strings allowed
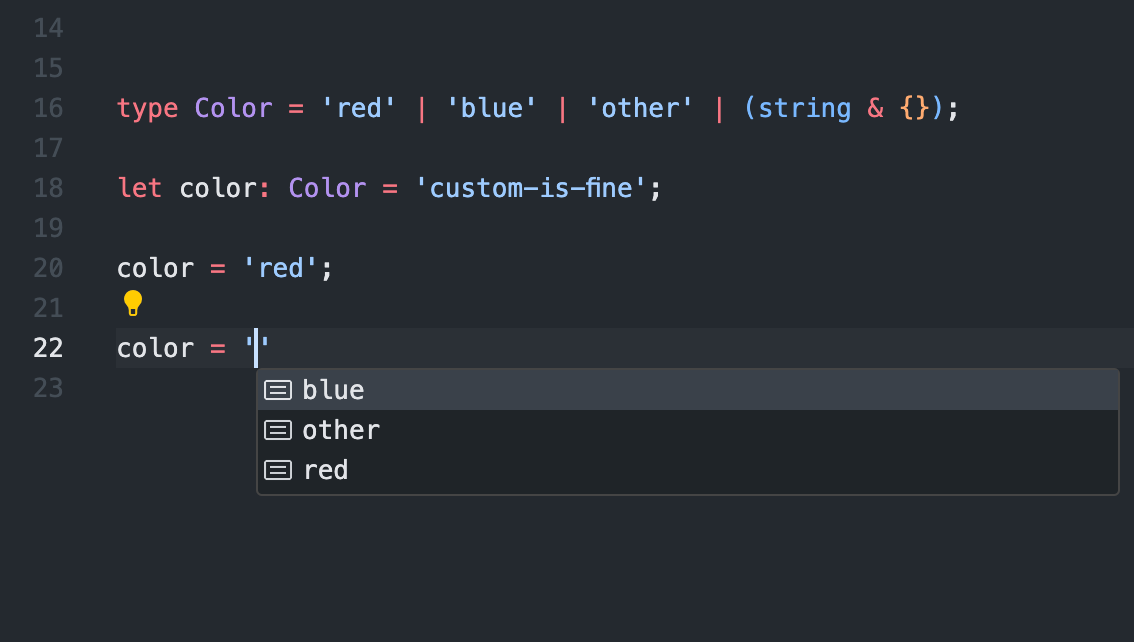
{}
Here is a TypeScript trick to keep IDE intellisense suggestions for known options but still allow arbitrary strings to be provided without any compiler errors.
As the main screenshot of this article shows, the solutions is as simple as that:
type Color = 'red' | 'blue' | 'other' | (string & {});
let color: Color = 'custom-is-fine';
color = 'red';
color = '' // here will be suggestions shown to choose from 'red' | 'blue' | 'other'
All the magic is done by (string & {})
in our union of options.
By providing string as intersection with & {}
we keep this string as separate option of union instead of collapsing the whole union to just any string.
This way autocomplete works by suggesting exact known literal options.
It is important to say that without intersection, our IDE autocompletions won't know what to suggest to write because union collapsed to just string as on the screenshot below:
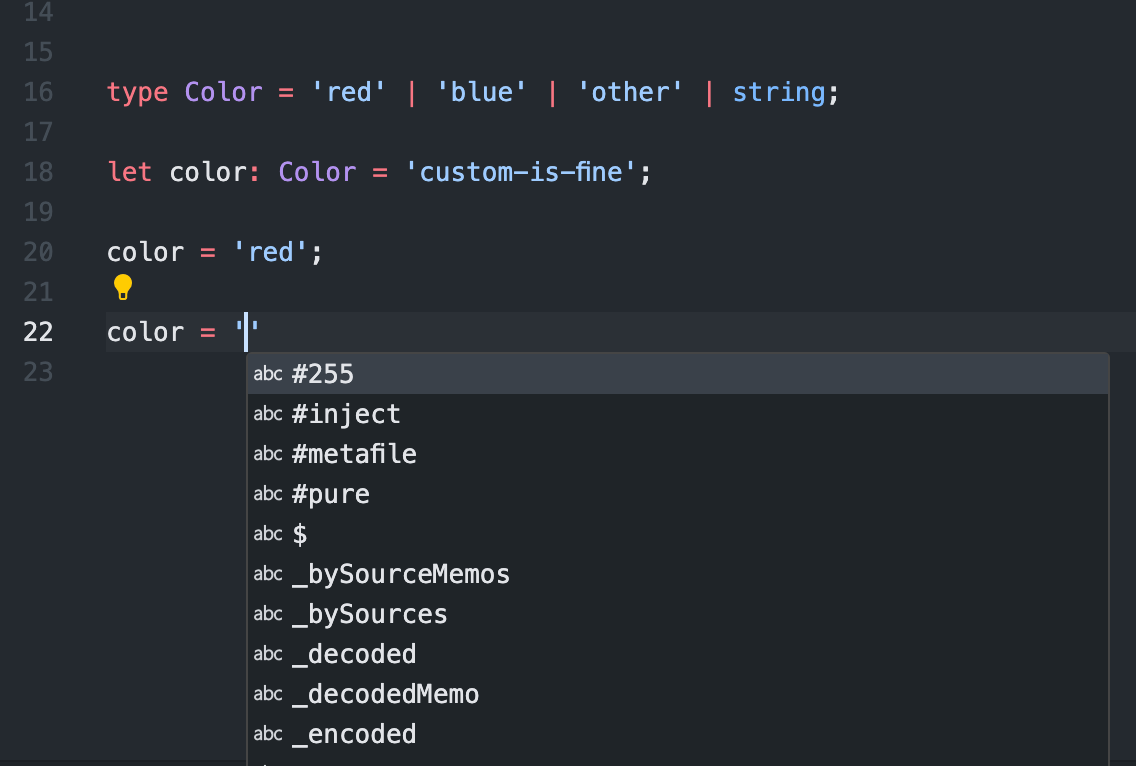
P.S. without string intersection in union, to open arbitrary suggestions for just a string press CMD+I in VS Code on Mac OS.
The trick was found during inspection of source code for cool tsup
package right on this line - https://github.com/egoist/tsup/blob/bee8f42dc72c5b40c2946422fc632069dde2a55b/src/options.ts#L94